Continuous Testing in DevOps: Tutorial & Best Practices
Continuous testing in DevOps represents an important shift in software development, emphasizing the integration of testing into every stage of the development lifecycle. This approach is vital to achieving the agility and efficiency required in modern software delivery. It enhances product quality and aligns closely with the principles of rapid, iterative development.
In this guide, we explore the essential best practices of continuous testing in a DevOps environment, offering insights into how teams can implement these strategies to streamline processes, improve software quality, and accelerate delivery timelines. As we delve into these practices, we focus on practical, actionable steps that can be taken to fully harness the benefits of continuous testing in the dynamic world of software development.
Summary of key concepts in continuous testing  in DevOps
The table below provides a list of the best practices discussed in this article.
Explanations of continuous testing best practices in DevOps
The following sections expand upon the best practices in the summary table above and give actionable recommendations for how to integrate continuous testing into DevOps environments.
Shorten the feedback loop
The essence of continuous testing in DevOps is encapsulated in the principle of shortening the feedback loop. The objective is to make the testing process not just a phase but an integral part of the development lifecycle. This approach ensures that any changes in the system are quickly evaluated and feedback is provided immediately.
To achieve this goal, teams must integrate testing into the earliest stages of development. Doing so can allow them to detect any flaws or issues so they can be promptly addressed, saving time and resources in the long run. Tools that facilitate continuous integration and delivery, such as Jenkins, Travis CI, CircleCI, and GitHub Actions play an important role in this process. They automate the testing process and provide real-time feedback to developers.
Furthermore, fostering a culture where developers and testers collaborate closely can be highly beneficial for teams. Regular meetings and effective communication channels ensure that feedback from testing is efficiently conveyed and acted upon. This collaboration not only streamlines the development process but also enhances the overall quality of the software.
Shift left
“Shifting left” in continuous testing refers to the practice of testing early and often in the software development lifecycle. By shifting the testing process to earlier stages of development, teams can detect and resolve issues before they become complex and costly to fix.
The diagram below shows the difference in effort throughout the software development lifecycle between shift left and traditional testing:
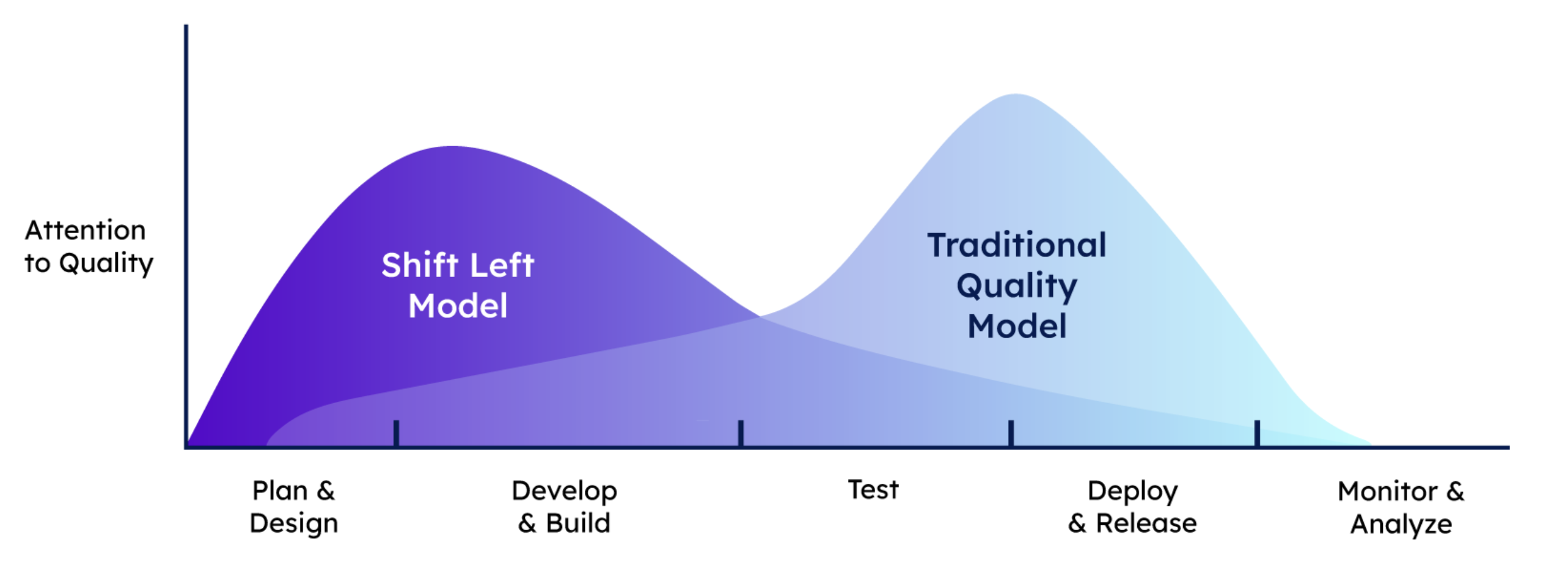
Shifting left can involve a fundamental change in the development team’s approach to testing. It requires integrating testing into every stage of software development, from requirements gathering to design, coding, and deployment. This approach ensures that testing is not an afterthought but a continuous activity parallel to development.
Test-driven development (TDD) and behavior-driven development (BDD) are instrumental in implementing the “shift left” approach.
Test-driven development is a software development approach where developers write code in three primary steps:
- Write a failing test for a new feature or function.
- Write a minimal amount of code to pass that test.
- Refactor the code for better design and to ensure it aligns with company and industry standards for cleanliness, readability, etc.
This process is then repeated for the next code change or new feature.
Behavior-driven development is a methodology that enhances communication among stakeholders in a project by describing the behavior of the software in readable and understandable language. This helps ensure that all parties involved have a clear understanding of the intended outcomes before any code is written.
Together, TDD and BDD encourage developers to clearly define expected behaviors and write tests before writing code, ensuring that each feature is thoroughly understood and tested as it is developed. This leads to a more robust and error-free codebase, reducing the likelihood of significant issues in later stages.
Automate where appropriate
Automation is a cornerstone of effective continuous testing. It involves identifying tasks within the testing process that can be automated to save time, reduce errors, and increase efficiency. The key is to automate repetitive and time-consuming tasks without undermining testing quality.
The choice of automation tools is critical. They should integrate seamlessly with the existing development environment and cater to the project’s needs. Popular tools include Cypress or Puppeteer for web automation, Espresso or XCTest for mobile application testing, and GitHub Actions or GitLab for orchestrating CI/CD pipelines.
However, it is essential to maintain a balance between automated and manual testing. While automation can speed up the process and handle repetitive tasks, manual testing is necessary for areas that require human judgment and intuition, such as usability and exploratory testing.
Improve environment parity
Maintaining environment parity refers to keeping the development, testing, and production environments as similar as possible. This similarity ensures the software behaves consistently across all development lifecycle stages, reducing the chances of encountering unexpected issues during deployment.
Achieving environment parity involves using tools and practices that standardize environments. Containerization tools like Docker help create consistent environments that are easily replicated across development, testing, and production.
Configuration management tools such as Ansible, Chef, or Puppet are vital in maintaining consistency across different environments. They automate configuring and maintaining servers, ensuring that all environments are set up identically.
Regular testing and monitoring of environments for parity help identify any discrepancies early on and allow the development team to address them before they impact the software deployment process or product performance.
Remember non-functional tests
While functional testing evaluates the software’s features and functionality, non-functional testing is equally important. It encompasses aspects like performance, security, reliability, usability, and compatibility, all of which are crucial for the overall quality of the software.
Integrating non-functional testing into the continuous testing process requires a strategic approach that involves selecting specialized tools for each type of non-functional test. For instance, tools like Multiple can be used for load and performance testing, while tools like ZAP or Nessus are suitable for security testing.
{{banner-2="/design/banners"}}
Using Multiple, we can create a performance test to validate the response time of an endpoint in an API. The test will follow the principles of shift left and shorten the feedback loop. The test script will be concise, executable across environments, and quick to run so that it integrates seamlessly into all CI/CD pipeline stages.
First, here is our test script:
There are a couple of key takeaways from this example:
- process.env.API_BASE_URL is reading our API URL. By reading the environment variable, we can quickly and easily target multiple environments in our CI/CD pipeline. For example, we can run staging and then immediately run a separate test against production simply by providing a different value for API_BASE_URL.
- The test targets only the /chat endpoint. It has one goal and does not involve chaining steps and transactions. For example, we did not need to POST, then GET, and then POST again. This isolation makes the tests more reliable because we know exactly what it means when there is a failure. It also results in much faster tests, which is key when testing in lower environments, like staging and development, where shorter feedback loops are needed.
- Multiple’s built-in Axios instance automatically captures response times for each request. In this case, it would provide metrics for POST /login, POST /chat, and GET /chat for each HTTP status code captured during the test.
- We recommend keeping the run time short (5-10 minutes) when running the tests. The goal for this type of test within continuous testing is to run often in all environments so we have a short feedback loop. You will want to schedule a longer-running performance test in a higher environment, such as production or pre-production, with more resources, running less often there since it consumes more resources and lengthens the feedback loop.
Regular updates and reviews of non-functional test cases are necessary to keep them relevant to changing requirements and technologies. In addition, gathering user feedback is vital for assessing the usability and compatibility of the software from an end-user perspective.
Integrate testing into the CI/CD pipeline
Integrating testing into each CI/CD pipeline phase ensures that any new changes are thoroughly vetted before deployment. This integration plays a critical role in maintaining the quality and stability of the software.
Designing a CI/CD pipeline that includes automated test execution at various stages helps prevent defects, allows for more agile development, and reduces the feedback loop for developers. This design includes testing after each build, before deployment, and after deployment. The pipeline should be designed to halt or roll back changes if tests fail, ensuring that only quality code is moved to the next stage.
A well-thought-out branching strategy is crucial for isolating changes and facilitating testing. When a test fails in isolation on a branch, it becomes easier to identify the offending code and owner by keeping the context separate from the application as a whole. Techniques like feature branching or Gitflow enable teams to test new features independently before merging them into the main codebase.
{{banner-1="/design/banners"}}
Final thoughts
Continuous testing in DevOps is a critical practice that intertwines closely with agile and efficient software development principles. By adopting best practices such as shortening the feedback loop, shifting testing left, judicious automation, maintaining environment parity, not overlooking non-functional tests, and integrating testing into the CI/CD pipeline, organizations can significantly enhance the quality, reliability, and speed of their software delivery.
These practices are not just procedural improvements but are fundamental to fostering a culture of continuous improvement and collaboration. As the technological landscape evolves, so does the software development approach. Continuous testing stands as a pillar supporting this evolution towards more resilient, user-centric, and high-quality software products.
‍